| | Homework: Java
This page provides details on assignment 3-1.
It is assigned in week 3 and
due in week 5. It is worth 150 points.
This is an individual assignment.
Outcomes
After completing this homework assignment, you will
have gained experience with
- Implementing a simple web application
- Simple XML processing
The assignment
Implement the RESTful interface below as a Java EE
web application.
More information
- your web application will be delivered as a single
WAR file
- your web application must work when deployed in
GlassFish
- your web application must implement the interface
defined below
- you may implement your servlet directly, or use JAX-RS,
or use some other Java EE compatible technology that seems appropriate, if
you are familiar with one
- you may use JAXB to
generate XML responses, or create the XML directly in your code.
A test suite for the Java homework
is available.
Interface
- Methods
- your application should support http GET, POST,
PUT and DELETE, according to the specifications below.
- XML
- Input content
- No request types have content. This is a bit of
"controlled unreality," intended to simplify the interface and
reduce work
- Output content
- Successful GET requests should return an XML
document, whose root is either a <student>
or <student-list>
element. See below for details.
- POST, PUT and DELETE should not return
any content. See below for their response codes.
- Parameters
- Your servlet should ignore request parameters
- Output headers
- The Content-type header on GET responses should be "text/xml;charset=utf-8"
Graphical view of grades.xsd
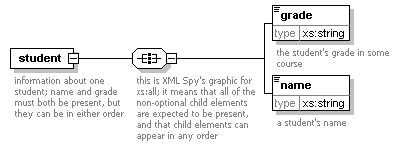
URIs
Form |
Used when |
/student |
Looking up all students
(with GET) |
/student/name |
Looking up a student
(with GET), removing a student (with DELETE) |
/student/name/grade/grade |
Adding a student and his/her grade, or updating
a student's grade (both can be done with POST or PUT) |
Back to top
Methods
GET
The homework servlet uses GET
requests for all lookups. The URIs used in GET requests are side-effect free, bookmark-able
and cacheable. The only reason for a change in the results of a GET request is
an update to the underlying content.
POST
The homework servlet uses POST
requests for creating or updating student records.
PUT
The homework servlet uses PUT
requests for the same purposes as POST: creating or updating student records.
DELETE
The directory service uses DELETE
requests for deleting student records.
Back to top
GET /student
The response is the complete list of students known
to the application.
Request content |
Response content |
Additional information |
none |
student-list
student*
name
grade
|
It is NOT an error if the servlet
doesn't know about any students. In that case, the student-list
element should be empty. |
Possible errors:
GET /student/name
The response is the information for the given
student, if any.
Request content |
Response content |
Additional information |
none |
student
name
grade
|
The student's name should be URL-encoded,
if necessary. For example, the URI /student/Jane%20Doe
should be used to request information
about Jane Doe. |
Possible errors:
- 404 - Not found; occurs if the application knows of no student with the given id.
Back to top
POST /student/name/grade/letter
Creates or updates a student record.
Request content |
Response content |
Additional information |
none
|
none
|
The student's name and letter
grade should be URL-encoded,
if necessary, for example, the URI /student/Jane%20Doe/grade/B%2B
means that Jane Doe received a B+ |
Possible errors:
- 400 - Bad request. Should be returned if letter
cannot be interpreted as a grade. Valid grades are the letters A, B, C,
D, E, F, I, W, and Z (upper or lower case). In addition, A-D (upper or
lower case) can be followed by + or - (not both). Examples of valid
grades: A, B-, b+, E, w, W. Examples of invalid grades: A!, f+, hello.
Back to top
PUT /student/name/grade/letter
Same as POST /student/name/grade/letter
Back to top
DELETE /student/name
Deletes the named student with the given id, if any.
Request content |
Response content |
Additional information |
none
|
none
|
The student's name should be URL-encoded,
if necessary. |
Possible errors:
- 404 - Not found; occurs if there is no student with the given id
Back to top
Deliverable: turn in a WAR file containing your web application. Specifically,
- Submit your WAR file to
the 655 drop box, which is accessible as Communications >> Drop Box on
the official materials page
- Submit your WAR file to
the drop box assignment called "3-1: Programming Homework".
- Your WAR file should be
named after you, with the .war suffix; for example, alice.war,
bob.war
- Your WAR file should be deployable in
GlassFish
- Your WAR file should include the Java source code for your servlet and the other classes you wrote, if
any. This is not a standard part of a web application, but it provides a convenient way for you to send me your source
code.
Include your source code in
WEB-INF/classes/,
along with your object code. If you're using Eclipse, it will do this for
you (check "Export source files" when you export your web project
as a WAR)
- Contents of your WAR file
File or
directory |
Contents |
WEB-INF/web.xml |
identifies your
servlet and servlet mapping |
WEB-INF/classes/ |
your source and
object
code |
- Testing and grading
- First, I will deploy your WAR file in GlassFish
- Then I will run the
homework test suite against your
service
- I may (or may not)
run additional tests. If I do, they will use only features defined in
the interface specification above.
|